Last updated on October 22nd, 2024
Entity to DTO conversion in a Spring Boot Application is common. We can use Manual Conversion or ModelMapper to convert an Entity to DTO and vice versa. In this topic, we will learn how to implement Entity to DTO conversion in Spring Boot using ModelMapper.

Table of content:
- Keep eclipse IDE ready(STS Integrated)
- Create a Spring Boot Starter Project
- Configure Model Mapper
- Define Database Connection in the application.properties file
- Create Entity class
- Create a Repository
- Create a Service
- Create a DTO class
- Create a Rest Controller class
- Run the Project
Model Mapper Dependency:
<dependency>
<groupId>org.modelmapper</groupId>
<artifactId>modelmapper</artifactId>
<version>2.3.8</version>
</dependency>
1. Keep eclipse IDE ready(STS Integrated)
Refer to this article How to Create Spring Project in IDE to create Spring Boot Project in Eclipse IDE.
2. Create a Spring Boot Starter Project
Add the following dependencies:
• Spring Web
• Spring Data JPA
• Mysql Driver
Maven Dependency
pom.xml:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.6.2</version>
<relativePath />
<!-- lookup parent from repository -->
</parent>
<groupId>com.demo</groupId>
<artifactId>Spring_Boot_Rest_ApiModel_Mapper</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>Spring_Boot_Rest_ApiModel_Mapper</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.modelmapper</groupId>
<artifactId>modelmapper</artifactId>
<version>2.3.8</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
3. Configure Model Mapper
package com.poc.rest;
import org.modelmapper.ModelMapper;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.Bean;
@SpringBootApplication
public class SpringBootRestApiModelMapperApplication {
public static void main(String[] args) {
SpringApplication.run(SpringBootRestApiModelMapperApplication.class, args);
}
@Bean
public ModelMapper modelMapper() {
return new ModelMapper();
}
}
4. Define Database Connection in the application.properties file
spring.datasource.url=jdbc:mysql://localhost:3306/user_db?useSSL=false
spring.datasource.username=root
spring.datasource.password=root
spring.jpa.hibernate.ddl-auto=update
spring.jpa.generate-ddl=true
spring.jpa.show-sql=true
5. Create Entity class
User.java:
package com.poc.rest.entity;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Integer id;
private String userName;
private String mobileNo;
private String emailId;
private String city;
private String password;
public User() {
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public String getMobileNo() {
return mobileNo;
}
public void setMobileNo(String mobileNo) {
this.mobileNo = mobileNo;
}
public String getEmailId() {
return emailId;
}
public void setEmailId(String emailId) {
this.emailId = emailId;
}
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
6. Create a Repository
UserRespository.java:
package com.poc.rest.repo;
import org.springframework.data.jpa.repository.JpaRepository;
import com.poc.rest.entity.User;
public interface UserRepos extends JpaRepository < User, Integer > {
}
7. Create a Service
UserService.java:
package com.poc.rest.service;
import java.util.List;
import com.poc.rest.entity.User;
public interface UserService {
public List < User > getAllUsersList();
public void save(User user);
public User findById(Integer id);
public void delete(User user);
}
UserServiceImp.java
package com.poc.rest.service;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.poc.rest.entity.User;
import com.poc.rest.repo.UserRepos;
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserRepos userRepo;
@Override
public List < User > getAllUsersList() {
return userRepo.findAll();
}
@Override
public void save(User user) {
userRepo.save(user);
}
@Override
public User findById(Integer id) {
return userRepo.findById(id).get();
}
@Override
public void delete(User user) {
userRepo.delete(user);
}
}
8. Create a DTO class
UserDTO.java:
package com.poc.rest.dto;
public class UserDTO {
private Integer id;
private String userName;
private String mobileNo;
private String emailId;
private String city;
private String password;
public UserDTO() {}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public String getMobileNo() {
return mobileNo;
}
public void setMobileNo(String mobileNo) {
this.mobileNo = mobileNo;
}
public String getEmailId() {
return emailId;
}
public void setEmailId(String emailId) {
this.emailId = emailId;
}
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
→ DTO: Data transfer objects are just data holders which are used to transport data between layers and tiers.
9. Create a Rest Controller class
UserController.java:
package com.poc.rest.controller;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import org.modelmapper.ModelMapper;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.DeleteMapping;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.PutMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import com.poc.rest.dto.UserDTO;
import com.poc.rest.entity.User;
import com.poc.rest.service.UserService;
@RestController
@RequestMapping("/api")
public class UserController {
@Autowired
private UserService userService;
@Autowired
private ModelMapper modelMapper;
@GetMapping("/users")
public ResponseEntity < ? > getUser() {
Map < String, Object > jsonResponseMap = new LinkedHashMap < String, Object > ();
List < User > listofUser = userService.getAllUsersList();
List < UserDTO > listofUserDto = new ArrayList < UserDTO > ();
if (!allUsers.isEmpty()) {
for (User user: listofUser ) {
listofUserDto.add(modelMapper.map(user, UserDTO.class));
}
jsonResponseMap.put("status", 1);
jsonResponseMap.put("data", listofUserDto);
return new ResponseEntity < > (jsonResponseMap, HttpStatus.OK);
} else {
jsonResponseMap.clear();
jsonResponseMap.put("status", 0);
jsonResponseMap.put("message", "Data is not found");
return new ResponseEntity < > (jsonResponseMap, HttpStatus.NOT_FOUND);
}
}
@PostMapping("/save")
public ResponseEntity < ? > saveUser(@RequestBody UserDTO userDto) {
Map < String, Object > jsonResponseMap = new LinkedHashMap < String, Object > ();
//convert DTO to an entity
User user = modelMapper.map(userDto, User.class);
userService.save(user);
jsonResponseMap.put("status", 1);
jsonResponseMap.put("message", "Record is Saved Successfully!");
return new ResponseEntity < > (jsonResponseMap, HttpStatus.CREATED);
}
@GetMapping("/user/{id}")
public ResponseEntity < ? > getUserById(@PathVariable Integer id) {
Map < String, Object > jsonResponseMap = new LinkedHashMap < String, Object > ();
try {
User user = userService.findById(id);
//convert entity to DTO
UserDTO userDto = modelMapper.map(user, UserDTO.class);
jsonResponseMap.put("status", 1);
jsonResponseMap.put("data", userDto);
return new ResponseEntity < > (jsonResponseMap, HttpStatus.OK);
} catch (Exception ex) {
jsonResponseMap.clear();
jsonResponseMap.put("status", 0);
jsonMap.put("message", "Data is not found");
return new ResponseEntity < > (jsonResponseMap, HttpStatus.NOT_FOUND);
}
}
@DeleteMapping("/delete/{id}")
public ResponseEntity < ? > deleteUser(@PathVariable Integer id) {
Map < String, Object > jsonResponseMap = new LinkedHashMap < String, Object > ();
try {
User user = userService.findById(id);
userService.delete(user);
jsonResponseMap.put("status", 1);
jsonResponseMap.put("message", "Record is deleted successfully!");
return new ResponseEntity < > (jsonResponseMap, HttpStatus.OK);
} catch (Exception ex) {
jsonResponseMap.clear();
jsonResponseMap.put("status", 0);
jsonResponseMap.put("message", "Data is not found");
return new ResponseEntity < > (jsonResponseMap, HttpStatus.NOT_FOUND);
}
}
@PutMapping("/update/{id}")
public ResponseEntity < ? > updateUser(@PathVariable Integer id, @RequestBody UserDTO userDto) {
Map < String, Object > jsonResponseMap = new LinkedHashMap < String, Object > ();
try {
User user = userService.findById(id);
user.setUserName(userDto.getUserName());
user.setMobileNo(userDto.getMobileNo());
user.setEmailId(userDto.getEmailId());
user.setCity(userDto.getCity());
user.setPassword(userDto.getPassword());
userService.save(user);
jsonMap.put("status", 1);
jsonResponseMap.put("data", userService.findById(id));
return new ResponseEntity < > (jsonResponseMap, HttpStatus.OK);
} catch (Exception ex) {
jsonResponseMap.clear();
jsonResponseMap.put("status", 0);
jsonResponseMap.put("message", "Data is not found");
return new ResponseEntity < > (jsonResponseMap, HttpStatus.NOT_FOUND);
}
}
}
10. Run the Project
POST Type: http://localhost:8080/api/save

GET Type: http://localhost:8080/api/users

GET Type: http://localhost:8080/api/user/3
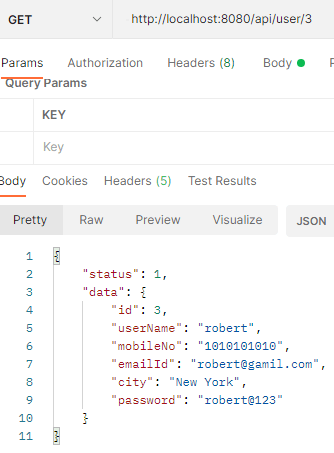
PUT Type: http://localhost:8080/api/update/3
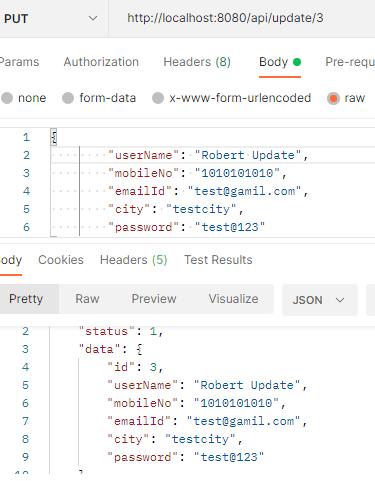
DELETE Type: http://localhost:8080/api/delete/3
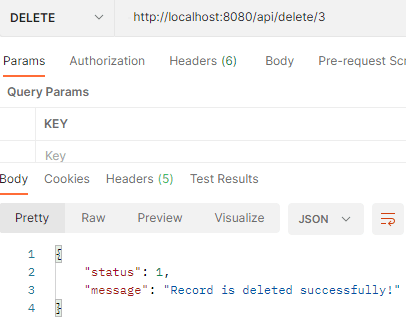
Conclusion:
This topic is explained What is a Model Mapper? How to use a Model Mapper? How to convert an Entity to DTO and vice versa? How to configure Model Mapper in Spring Boot Rest API App?
good for working people live project problem solving topics