In this topic, we will send mail via SMTP using JavaMail Library. We will create step-by-step Restful web services that can send an email with or without attachments. We will learn to generate app passwords in Gmail.
Table of content
1. How to generate app passwords in Gmail?
2. Create Spring Boot Project using eclipse IDE integrated STS plugin
2.1 Keep eclipse IDE ready
2.2 Create a Spring Boot Starter Project
2.3 Configure the required configurations in the application.properties file
2.4 Create a Bean class
2.5 Create Service
2.6 Create a RestController class
2.7 Run the Project
3. Conclusion
1. How to generate app passwords in Gmail?
We have to login to Gmail with our Gmail account credentials then we need to do 2-step verification enabled in our account for app password generation. For this, we have to follow these steps are:
1. Login to Gmail
2. Manage our Google Account
3. Security
4. App Passwords
5. Provide our login password
6. Select an app with a custom name
7. Click on Generate
2. Create Spring Boot Project using eclipse IDE integrated STS plugin
2.1 Keep eclipse IDE ready
2.2 Create a Spring Boot Starter Project
Please Select Spring Web and Java Mail Sender dependencies for the project.
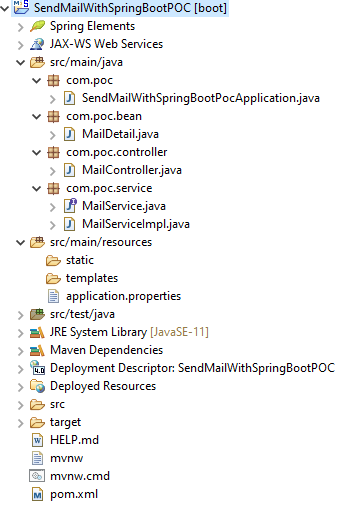
SpringBootApplication Class:
package com.poc;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class SendMailWithSpringBootPocApplication {
public static void main(String[] args) {
SpringApplication.run(SendMailWithSpringBootPocApplication.class, args);
}
}
pom.xml:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.6.3</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.poc</groupId>
<artifactId>SendMailWithSpringBootPOC</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>war</packaging>
<name>SendMailWithSpringBootPOC</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>11</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
2.3 Configure the required configurations in the application.properties file
spring.mail.host=smtp.gmail.com
spring.mail.username=<Login User to SMTP server>
spring.mail.password=<app password>
spring.mail.properties.mail.smtp.auth=true
# TLS, port 587
spring.mail.port=587
spring.mail.properties.mail.smtp.starttls.enable=true
server.port=8888
# SSL, port 465
#spring.mail.properties.mail.smtp.socketFactory.port = 465
#spring.mail.properties.mail.smtp.socketFactory.class = javax.net.ssl.SSLSocketFactory
→ In this file we configure the required configuration of the Gmail SMTP server.
2.4 Create a Bean class
MailDetail.java:
package com.poc.bean;
public class MailDetail {
private String recipient;
private String subject;
private String msgBody;
private String attachment;
public MailDetail() {
}
public String getRecipient() {
return recipient;
}
public void setRecipient(String recipient) {
this.recipient = recipient;
}
public String getSubject() {
return subject;
}
public void setSubject(String subject) {
this.subject = subject;
}
public String getMsgBody() {
return msgBody;
}
public void setMsgBody(String msgBody) {
this.msgBody = msgBody;
}
public String getAttachment() {
return attachment;
}
public void setAttachment(String attachment) {
this.attachment = attachment;
}
}
2.5 Create Service
MailService.java:
package com.poc.service;
import com.poc.bean.MailDetail;
public interface MailService {
String sendMail(MailDetail mailDetail);
String sendMailWithAttachment(MailDetail mailDetail);
}
MailServiceImpl.java:
package com.poc.service;
import java.io.File;
import javax.mail.MessagingException;
import javax.mail.internet.MimeMessage;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.core.io.FileSystemResource;
import org.springframework.mail.SimpleMailMessage;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.mail.javamail.MimeMessageHelper;
import org.springframework.stereotype.Service;
import com.poc.bean.MailDetail;
@Service
public class MailServiceImpl implements MailService {
@Autowired
private JavaMailSender mailSender;
@Value("${spring.mail.username}")
private String sender;
@Override
public String sendMail(MailDetail mailDetail) {
// Try block to check for exceptions handling
try {
// Creating a simple mail message object
SimpleMailMessage emailMessage = new SimpleMailMessage();
// Setting up necessary details of mail
emailMessage.setFrom(sender);
emailMessage.setTo(mailDetail.getRecipient());
emailMessage.setSubject(mailDetail.getSubject());
emailMessage.setText(mailDetail.getMsgBody());
// Sending the email
mailSender.send(emailMessage);
return "Email has been sent successfully...";
}
// Catch block to handle the exceptions
catch (Exception e) {
return "Error while Sending email!!!";
}
}
@Override
public String sendMailWithAttachment(MailDetail mailDetail) {
// Creating a Mime Message
MimeMessage mimeMessage = mailSender.createMimeMessage();
MimeMessageHelper mimeMessageHelper;
try {
// Setting multipart as true for attachment to be send
mimeMessageHelper = new MimeMessageHelper(mimeMessage, true);
mimeMessageHelper.setFrom(sender);
mimeMessageHelper.setTo(mailDetail.getRecipient());
mimeMessageHelper.setSubject(mailDetail.getSubject());
mimeMessageHelper.setText(mailDetail.getMsgBody());
// Adding the file attachment
FileSystemResource file = new FileSystemResource(new File(mailDetail.getAttachment()));
mimeMessageHelper.addAttachment(file.getFilename(), file);
// Sending the email with attachment
mailSender.send(mimeMessage);
return "Email has been sent successfully...";
}
// Catch block to handle the MessagingException
catch (MessagingException e) {
// Display message when exception is occurred
return "Error while sending email!!!";
}
}
}
→ JavaMailSender interface of JavaMail API is used here to send simple text mail.
→ To send a more sophisticated mail with an attachment, MimeMessage can be used. MimeMessageHelper works as a helper class for MimeMessage to add the attachment and other details required to send the mail message.
2.6 Create a RestController class
MailController.java:
package com.poc.controller;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import com.poc.bean.MailDetail;
import com.poc.service.MailService;
@RestController
@RequestMapping("/api")
public class MailController {
@Autowired
private MailService mailService;
//Sending email
@PostMapping("/send-mail")
public String sendMail(@RequestBody MailDetail mailDetail) {
return mailService.sendMail(mailDetail);
}
//Sending email with attachment
@PostMapping("/send-mail-attachment")
public String sendMailWithAttachment(@RequestBody MailDetail mailDetail)
{
return mailService.sendMailWithAttachment(mailDetail);
}
}
2.7 Run the Project
Right-click on the main class (SendMailWithSpringBootPocApplication.java) then click on Run As after that select Java Application.
Hitting this URL “http://localhost:8888/api/send-mail” on the Postman
Mail Received on the Gmail:
Hitting this URL “http://localhost:8888/api/send-mail-attachment” on the Postman
Mail Received on the Gmail:
3. Conclusion
In this topic, we learned about generating app passwords in Gmail, creating Restful web services and sending mail in Spring Boot using JavaMail Library.