Last updated on May 17th, 2024
In this example, we create a form for user input, and a radio button and show user details through the Spring MVC form tag.
<form:radiobutton path="gender"/>
This tag is provided by the Spring MVC form tag for the radio button.

In this example of an application, we can implement a radio button Spring MVC form tag. We will make a form layout i.e., “user-form.jsp”. In this form, we take two input fields first for the first name entering the field and a second field for the last name entering the field. We will take two radio buttons one for the male radio button and the other for the female radio button.
We will take one button for submitting the form data. The User class is a bean class that contains firstName,lastName, and gender properties.
UserController is a Java class that is annotated with the “@Controller” annotation to make the controller class of this application.
Development Process:
1. Keep Eclipse IDE ready
2. Project Structure
3. Add the jar file
4. Configure Spring DispacherServlet
5. Add configuration to a file
6. Create View Page
7. Create Bean Class
8. Create Controller Class
9. Run the App
1. Keep Eclipse IDE ready
2. Project Structure

3. Add the jar file
common-logging-<version>.jar
spring-aop-<version>.jar
spring-context-<version>.jar
spring-core-<version>.jar
spring-expression-<version>.jar
spring-web-<version>.jar
spring-webmvc-<version>.jar
4. Create a View Page
home.jsp:
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%>
<!DOCTYPE html>
<html>
<head>
<body>
<a href="http://localhost:8080/spring_mvc_radio_button_demo/user/showForm" >Click For User Form</a>
</body>
</html>
user-form.jsp:
<%@ taglib prefix="form" uri="http://www.springframework.org/tags/form"%>
<!DOCTYPE html>
<html>
<head>
<title>User Registration Form</title>
</head>
<body>
<form:form action="processForm" modelAttribute="user">
First name: <form:input path="firstName" />
<br>
<br>
Last name: <form:input path="lastName" />
<br>
<br>
Gender:<br>
<form:radiobutton path="gender" value="Male"/>Male
<form:radiobutton path="gender" value="Female"/>Female
<br>
<br>
<input type="submit" value="Submit" />
</form:form>
</body>
</html>
→ <form:radiobutton path=”gender”/> “gender” is a bean class property name.
user-detail.jsp:
<!DOCTYPE html>
<html>
<head>
<title>User Detail</title>
</head>
<body>User Detail: ${user.firstName} ${user.lastName} Gender-${user.gender}
</body>
</html>
5. Configure Spring DispacherServlet
web.xml:
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4.xsd"id="WebApp_ID" version="4.0">
<display-name>spring-mvc-radio-button-demo</display-name>
<absolute-ordering />
<!-- Spring MVC Configs -->
<!-- Configure Spring MVC Dispatcher Servlet -->
<servlet>
<servlet-name>dispatcher</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/dispatcher-servlet.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>dispatcher</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
</web-app>
→ This file is created at the “WEB-INF” directory in the application of radio button example POC(Proof of Concept).
6. Add configuration to file
dispatcher-servlet.xml:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc.xsd">
<!-- Add support for component scanning -->
<context:component-scan base-package="com.poc" />
<!-- Add support for conversion, formatting and validation support -->
<mvc:annotation-driven/>
<!-- Define Spring MVC view resolver -->
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/view/" />
<property name="suffix" value=".jsp" />
</bean>
</beans>
7. Create Bean Class
User.java:
package com.poc.controller.bean;
public class User {
private String firstName;
private String lastName;
private String gender;
public User() {
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
}
8. Create Controller Class
HomeController.java:
package com.poc.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class HomeController {
@RequestMapping("/")
public String showPage() {
return "home";
}
}
UserController.java:
package com.poc.controller;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import com.poc.controller.bean.User;
@Controller
@RequestMapping("/user")
public class UserController{
@RequestMapping("/showForm")
public String showForm(Model theModel) {
// create a User object
User user=new User();
theModel.addAttribute("user",user);
return "user-form";
}
@RequestMapping("/processForm")
public String processForm(@ModelAttribute("user") User user) {
return "user-detail";
}
}
9. Run the App
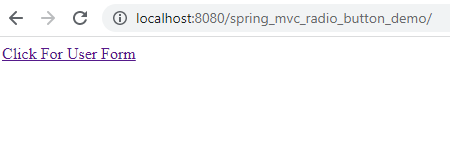
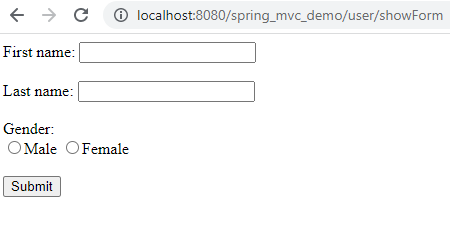

Conclusion:
This example is explained What is a radio tag in the Spring MVC form tag library? How to use <form:radiobutton /> tag?