In this example, we can export data into PDF in Spring Boot from the H2 database. To achieve this task we can use the OpenPDF library.

Configuring OpenPDF library
This dependency will be configured in the pom.xml of the project.
<dependency>
<groupId>com.github.librepdf</groupId>
<artifactId>openpdf</artifactId>
<version>1.3.26</version>
</dependency>
Table of contents
1. Creating Spring Boot Project
2. Keep Eclipse IDE ready
3. Defining Configuration
4. Creating a JPA Entity class
5. Creating a JPA Repository
6. Creating Service
7. Creating a PDF file Generator class
8. Creating a Controller class
9. Creating a view page
10. Inserting Records
11. Run the Spring Boot Project
1. Creating Spring Boot Project
We are creating a Spring Boot Project from the web tool Spring Initializr or you can use IDE.
Select the following dependencies:
• Spring Web
• Spring Data JPA
• H2 Database
2. Keep Eclipse IDE ready
Importing the spring boot project in the IDE which you created for this example.
Project Structure of Export data into PDF in Spring Boot Project

Maven Dependency
pom.xml
Here is the complete pom.xml file of the project.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.6.3</version>
<relativePath /> <!-- lookup parent from repository -->
</parent>
<groupId>com.example</groupId>
<artifactId>Export_PDF_Spring_Boot_Example</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>Export_PDF_Spring_Boot_Example</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>11</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>com.github.librepdf</groupId>
<artifactId>openpdf</artifactId>
<version>1.3.26</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
3. Defining Configuration
We are defining the H2 database configuration, embedded Tomcat server port and context path of the project.
#H2 Database
spring.datasource.url=jdbc:h2:mem:testdb
spring.datasource.driverClassName=org.h2.Driver
spring.datasource.username=admin
spring.datasource.password=
spring.jpa.database-platform=org.hibernate.dialect.H2Dialect
spring.h2.console.enabled=true
spring.jpa.show-sql=true
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.H2Dialect
spring.jpa.hibernate.ddl-auto= update
server.port=8888
server.servlet.context-path= /demo
4. Creating a JPA Entity class
We are creating a JPA entity class Student with these properties(id, studentName, email, mobileNo).
Student.java
package com.example.entity;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class Student {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private long id;
private String studentName;
private String email;
private String mobileNo;
public Student() {
}
public long getId() {
return id;
}
public void setId(long id) {
this.id = id;
}
public String getStudentName() {
return studentName;
}
public void setStudentName(String studentName) {
this.studentName = studentName;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getMobileNo() {
return mobileNo;
}
public void setMobileNo(String mobileNo) {
this.mobileNo = mobileNo;
}
}
5. Creating a JPA Repository
We are a JPA Repository to uses the default persistence methods(save(), findAll(), etc.). This JpaRepository is available in the spring data jpa.
StudentRepository.java
package com.example.repo;
import org.springframework.data.jpa.repository.JpaRepository;
import com.example.entity.Student;
public interface StudentRepoPDF extends JpaRepository<Student, Long> {}
6. Creating Service
We are a service interface StudentService with these method declarations (addStudent(-) and getStudentList(-)).
StudentService.java
package com.example.service;
import java.util.List;
import com.example.entity.Student;
public interface StudentService {
void addStudent(Student student);
List<Student> getStudentList();
}
We are creating an implementation class of the Service interface to implement all methods of it.
StudentServiceImpl.java
package com.example.service;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.example.entity.Student;
import com.example.repo.StudentRepoPDF;
@Service
public class StudentServiceImpl implements StudentService {
@Autowired
StudentRepoPDF studentRepo;
@Override
public void addStudent(Student student) {
studentRepo.save(student);
}
@Override
public List<Student> getStudentList() {
return studentRepo.findAll();
}
}
7. Creating a PDF file Generator class
We are creating a class which generates PDF file for this project.
PdfGenerator.java
package com.example.util;
import java.io.IOException;
import java.util.List;
import javax.servlet.http.HttpServletResponse;
import com.example.entity.Student;
import com.lowagie.text.Document;
import com.lowagie.text.DocumentException;
import com.lowagie.text.Font;
import com.lowagie.text.FontFactory;
import com.lowagie.text.PageSize;
import com.lowagie.text.Paragraph;
import com.lowagie.text.Phrase;
import com.lowagie.text.pdf.CMYKColor;
import com.lowagie.text.pdf.PdfPCell;
import com.lowagie.text.pdf.PdfPTable;
import com.lowagie.text.pdf.PdfWriter;
public class PdfGenerator {
public void generate(List < Student > studentList, HttpServletResponse response) throws DocumentException, IOException {
// Creating the Object of Document
Document document = new Document(PageSize.A4);
// Getting instance of PdfWriter
PdfWriter.getInstance(document, response.getOutputStream());
// Opening the created document to change it
document.open();
// Creating font
// Setting font style and size
Font fontTiltle = FontFactory.getFont(FontFactory.TIMES_ROMAN);
fontTiltle.setSize(20);
// Creating paragraph
Paragraph paragraph1 = new Paragraph("List of the Students", fontTiltle);
// Aligning the paragraph in the document
paragraph1.setAlignment(Paragraph.ALIGN_CENTER);
// Adding the created paragraph in the document
document.add(paragraph1);
// Creating a table of the 4 columns
PdfPTable table = new PdfPTable(4);
// Setting width of the table, its columns and spacing
table.setWidthPercentage(100 f);
table.setWidths(new int[] {3,3,3,3});
table.setSpacingBefore(5);
// Create Table Cells for the table header
PdfPCell cell = new PdfPCell();
// Setting the background color and padding of the table cell
cell.setBackgroundColor(CMYKColor.BLUE);
cell.setPadding(5);
// Creating font
// Setting font style and size
Font font = FontFactory.getFont(FontFactory.TIMES_ROMAN);
font.setColor(CMYKColor.WHITE);
// Adding headings in the created table cell or header
// Adding Cell to table
cell.setPhrase(new Phrase("ID", font));
table.addCell(cell);
cell.setPhrase(new Phrase("Student Name", font));
table.addCell(cell);
cell.setPhrase(new Phrase("Email", font));
table.addCell(cell);
cell.setPhrase(new Phrase("Mobile No", font));
table.addCell(cell);
// Iterating the list of students
for (Student student: studentList) {
// Adding student id
table.addCell(String.valueOf(student.getId()));
// Adding student name
table.addCell(student.getStudentName());
// Adding student email
table.addCell(student.getEmail());
// Adding student mobile
table.addCell(student.getMobileNo());
}
// Adding the created table to the document
document.add(table);
// Closing the document
document.close();
}
}
→ We can create a document by the defining page size of the document through the Document class.
→ We are getting a PdfWriter instance by providing the created document and OutputStream.
→ Creating paragraphs, tables, headings etc.
8. Creating a Controller class
We are creating a controller class which contains a request URL.
StudentController.java
package com.example.controller;
import java.io.IOException;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.List;
import javax.servlet.http.HttpServletResponse;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import com.example.entity.Student;
import com.example.service.StudentService;
import com.example.util.PdfGenerator;
import com.lowagie.text.DocumentException;
@Controller
public class StudentController {
@Autowired
private StudentService studentService;
@GetMapping("/export-to-pdf")
public void generatePdfFile(HttpServletResponse response) throws DocumentException, IOException
{
response.setContentType("application/pdf");
DateFormat dateFormat = new SimpleDateFormat("YYYY-MM-DD:HH:MM:SS");
String currentDateTime = dateFormat.format(new Date());
String headerkey = "Content-Disposition";
String headervalue = "attachment; filename=student" + currentDateTime + ".pdf";
response.setHeader(headerkey, headervalue);
List < Student > listofStudents = studentService.getStudentList();
PdfGenerator generator = new PdfGenerator();
generator.generate(listofStudents, response);
}
}
9. Creating a view page
We are creating a view page under the static folder of this project.
index.html
<!DOCTYPE html>
<html>
<head>
<title>Export PDF File</title>
</head>
<body class="container">
<p>Click the button for export PDF file.</p>
<a href="http://localhost:8888/demo/export-to-pdf" class="btn btn-primary">Download PDF File</a>
</body>
</html>
10. Inserting Records
We are inserting records in the H2 database table with the help of the CommandLineRunner interface.
package com.example;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import com.example.entity.Student;
import com.example.service.StudentService;
@SpringBootApplication
public class ExportPdfSpringBootExampleApplication implements CommandLineRunner {
@Autowired
private StudentService studentService;
public static void main(String[] args) {
SpringApplication.run(ExportPdfSpringBootExampleApplication.class, args);
}
@Override
public void run(String...a) {
for (int i = 0; i <= 10; i++) {
Student student = new Student();
student.setStudentName("Student Name");
student.setEmail("student@mail.com");
student.setMobileNo("XXXXXXXXXX");
studentService.addStudent(student);
}
}
}
11. Run the Spring Project
Right-click on the SpringBootApplication class(ExportPdfSpringBootExampleApplication), then click Run as Java Application. To check the database console. We can type this URL on the browser “http://localhost:8888/demo/h2-console/” for testing this example of whether the application created a table on the H2 database and whether data is inserted properly or not.
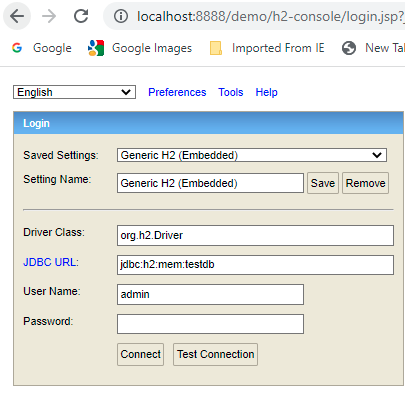

To check we can type this URL on our browser “http://localhost:8888/demo/” to test if this application is properly exporting data to pdf.

Click on the Download PDF File link to download the PDF file.
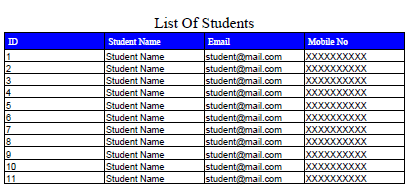
Conclusion
This example is explained:
• How to transform data into a pdf file from a database?
• How to use the openPDF library?