In this example, we can make the rest API in the Spring Boot(JPA+Mysql) project. Rest API helps to communicate with other technologies in an easy manner. Rest API gives response as JSON form output. In this Rest API CRUD(Create, Read, Update, and Delete) Operation POC(Proof of Concept) when we hit a particular URL then the request goes to the RestController class which executes the match request handler method. In the method call a particular service’s class method. The service method is executed and calls the predefined persistence method of Repository then the particular SQL query is executed on the database to perform the persistence operation. Finally, we will get a response in JSON format.
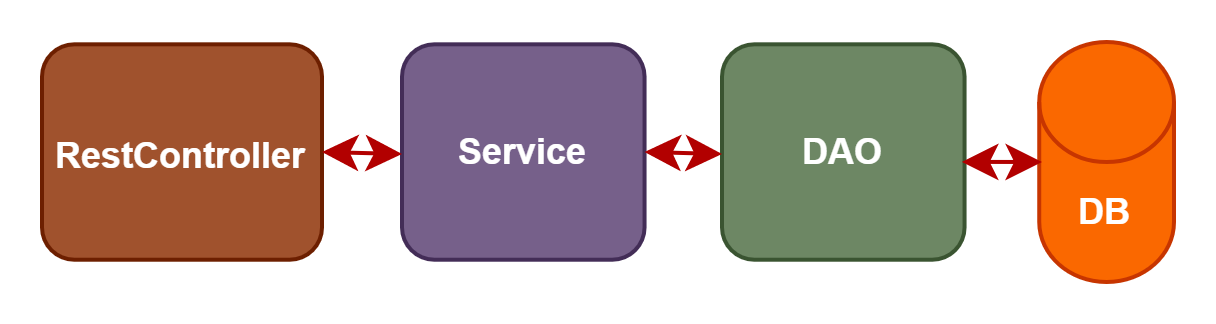
In this example we can create a user table using Java Code and Insert, Update, Select and Delete operations performing through java code.
In this example of the application, we will define database configuration. We will create one Java Bean class i.e., User.java.This class is annotated with @Entity annotation. Then this entity class is creating the table in the database through Java code. We will create one Repository interface that extends JpaRepository i.e., UserRespository.java which contains persistence predefined methods. We will create one service interface i.e., UserService.java which contains some methods. We will create a service class i.e., UserServiceImpl.java that implements the UserService interface which overrides all the methods which are declared in the UserService interface. This class is annotated with @Service annotation that’s why we call this class a Service class. This class is auto-wiring the UserRespository because we call all the predefined methods like save(),findById(), etc. The persistence methods trigger SQL queries in the background there is no need to write SQL by ourselves. We can manage these persistent SQL queries for this CRUD operation through Java code with the help of JPA. The JPA is very helpful for our applications. We will create a RestController class i.e., UserController which is annotated with @RestController.This class contains all the request handler methods. This class is auto-wiring the service interface for the call persistence methods which are defined in the service class that is implementing the service interface. This RestController class is delegated to the service layer because there are no business logic methods defined in it.
Table of content
1. Keep eclipse IDE ready
2. Create a Spring Boot Starter Project for the example of the CRUD REST API(Select Spring Web, Spring Data Jpa, Mysql Driver dependencies, and Select war)
3. Define Database Connection in application.properties
4. Create Entity class
5. Create DAO
6. Create a Service
7. Create a Rest Controller class
8. Run the Project
1. Keep eclipse IDE ready
2. Create a Spring Boot Starter Project for the example of the CRUD REST API(Select Spring Web, Spring Data Jpa, Mysql Driver dependencies, and Select war)
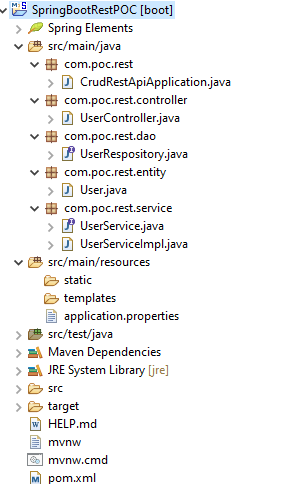
3. Define Database Connection in application.properties file
application.properties
spring.datasource.url=jdbc:mysql://localhost:3306/user_db?useSSL=false
spring.datasource.username=root
spring.datasource.password=root
spring.jpa.hibernate.ddl-auto=update
spring.jpa.generate-ddl=true
spring.jpa.show-sql=true
→ There is no need to define the driver class name “spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver” explicitly.This is automatically registered.
4. Create Entity class
User.java
package com.poc.rest.entity;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Integer id;
private String userName;
private String mobileNo;
private String emailId;
private String city;
private String password;
public User() {
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public String getMobileNo() {
return mobileNo;
}
public void setMobileNo(String mobileNo) {
this.mobileNo = mobileNo;
}
public String getEmailId() {
return emailId;
}
public void setEmailId(String emailId) {
this.emailId = emailId;
}
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
5. Create DAO
UserRespository.java
package com.poc.rest.dao;
import org.springframework.data.jpa.repository.JpaRepository;
import com.poc.rest.entity.User;
public interface UserRespository extends JpaRepository<User, Integer> {
}
6. Create a Service
UserService.java
package com.poc.rest.service;
import java.util.List;
import com.poc.rest.entity.User;
public interface UserService {
public List<User> getUser();
public void save(User user);
public User findById(Integer id);
public void delete(User user);
}
UserServiceImpl.java
package com.poc.rest.service;
import java.util.List;
import java.util.Optional;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.poc.rest.dao.UserRespository;
import com.poc.rest.entity.User;
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserRespository userRepo;
@Override
public List<User> getUser() {
return userRepo.findAll();
}
@Override
public void save(User user) {
userRepo.save(user);
}
@Override
public User findById(Integer id) {
Optional<User> userResult = userRepo.findById(id);
User user = null;
if (userResult.isPresent()) {
user = userResult.get();
}
return user;
}
@Override
public void delete(User user) {
userRepo.delete(user);
}
}
7. Create a Rest Controller class
UserController.java
package com.poc.rest.controller;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.DeleteMapping;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.PutMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import com.poc.rest.entity.User;
import com.poc.rest.service.UserService;
@RestController
@RequestMapping("/api")
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/users")
public List<User> getUser() {
return userService.getUser();
}
@PostMapping("/save")
public User saveUser(@RequestBody User user) {
userService.save(user);
return user;
}
@GetMapping("/user/{id}")
public User getUserById(@PathVariable Integer id) {
User user = userService.findById(id);
return user;
}
@DeleteMapping("/delete/{id}")
public User deleteUser(@PathVariable Integer id) {
User user = userService.findById(id);
userService.delete(user);
return user;
}
@PutMapping("/update/{id}")
public User updateUser(@PathVariable Integer id, @RequestBody User userDetail) {
User user = userService.findById(id);
user.setUserName(userDetail.getUserName());
user.setMobileNo(userDetail.getMobileNo());
user.setEmailId(userDetail.getEmailId());
user.setCity(userDetail.getCity());
user.setPassword(userDetail.getPassword());
userService.save(user);
return user;
}
}
8. Run the Project
You can test API through “Postman” Post Type: http://localhost:8080/api/save
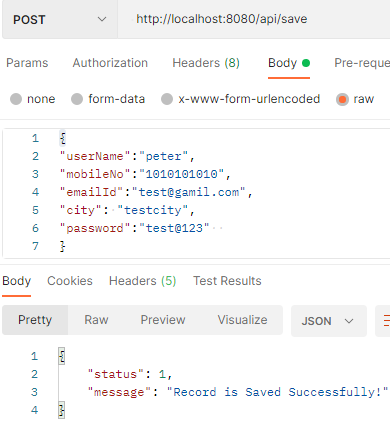
See the console when we hit this URL “http://localhost:8080/api/save” through postman insert query is triggered.
Get Type: http://localhost:8080/api/users
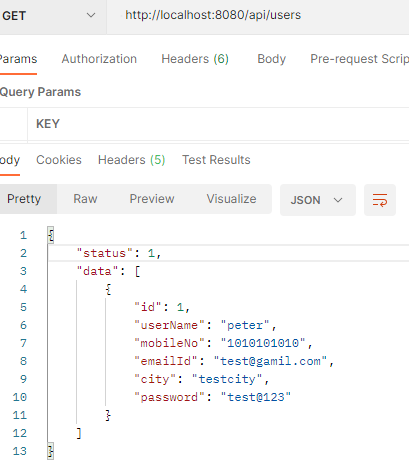
See the console when we hit this URL “http://localhost:8080/api/users” through postman select query is triggered.
Get Type: http://localhost:8080/api/user/1

See the console when we hit this URL “http://localhost:8080/api/user/1” through the postman select query with where clause is triggered.
Put Type: http://localhost:8080/api/update/1
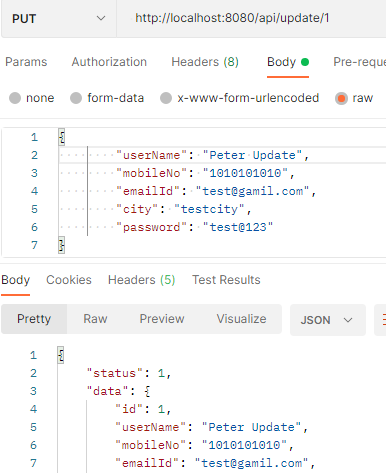
Delete Type: http://localhost:8080/api/delete/1
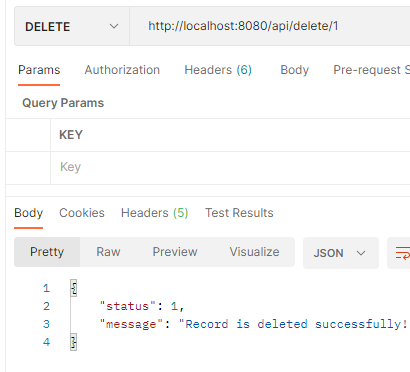
See the console when we hit this URL “http://localhost:8080/api/delete/1” through postman delete query is triggered.
Conclusion
This example is explained:
• How to create a Rest API in Spring Boot App?
• How to configure the MySql database in this App?
• How can we test Rest API in the Postman?