Last updated on April 3rd, 2024
In this topic, we will learn how to use Lombok in the Spring Boot Project step-by-step. To use this in our Spring Boot project we need to add Lombok dependency on it. We need to install Lombok and integrate with IDE to use feature this dependency. Let’s learn what is Lombok, what its use of it and how to install and integrate with Eclipse IDE.
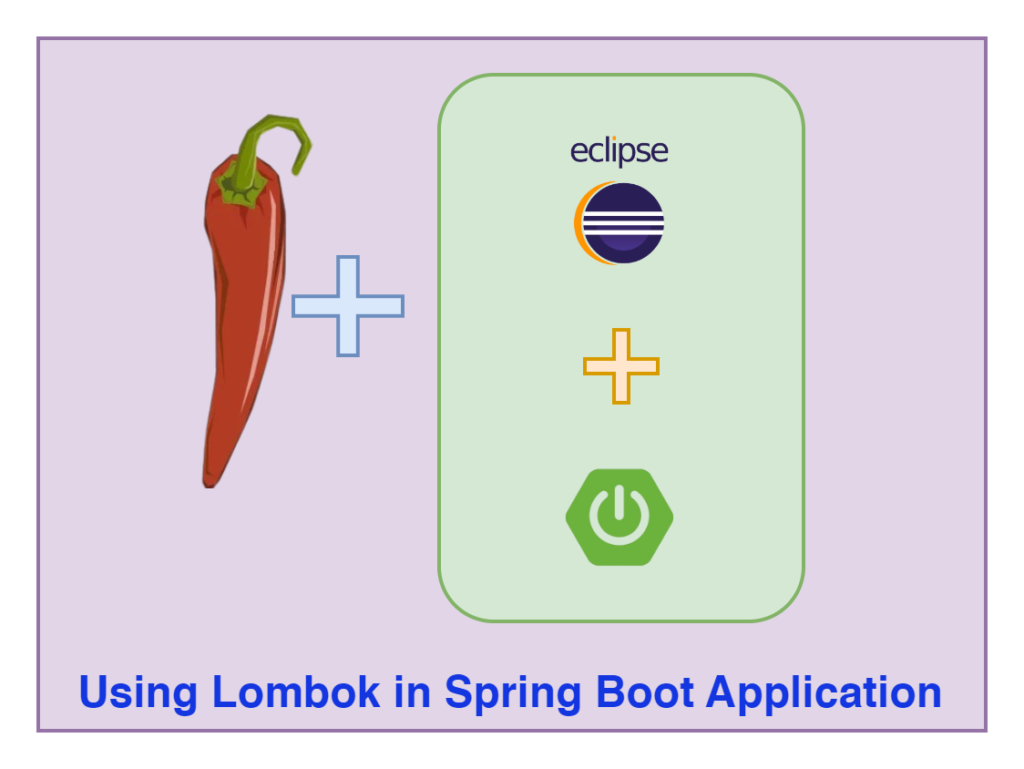
What is the Lombok?
Java Bean class and POJO class with some properties and their setter method, getter method, no parameterized constructor or parameterized constructor, toString method we can explicitly create in the class. Creating these things for the class is a time-consuming process while developing applications. But Lombok provides a solution to this problem of the POJO class and Java Bean. We don’t need to create a setter, getter of properties, no parameterized constructor or parameterized constructor, or toString method of this class explicitly by using this Lombok. This is the most popular Java library that minimizes or removes boilerplate code by using annotations.
Without Lombok of the POJO class
We are creating a Person.java class without using Lombok annotation.
Person.java
public class Person {
private Long id;
private String fName;
private String lName;
//zero param constructor
public Person(){
}
//getter of the id property
public Long getId() {
return id;
}
//setter of the id property
public void setId(Long id) {
this.id = id;
}
//getter of the first name property
public String getFName() {
return name;
}
//setter of the first name property
public void setFName(String name) {
this.name = name;
}
//getter of the last name property
public String getLName() {
return name;
}
//setter of the last name property
public void setLName(String name) {
this.name = name;
}
}
With Lombok of the POJO class
We are creating the same class Person.java with Lombok annotations.
Person.java
@NoArgsConstructor
public class Person {
@Getter
@Setter
private Long id;
@Getter
@Setter
private String fName;
@Getter
@Setter
private String lName;
}
How to Add Lombok?
To add Lombok in the Maven dependency we need to add this below dependency XML into the pom.xml for the Spring Boot project or we can add dependency while creating a Spring Boot Project through the Spring Initializr web tool.
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
How to install the Lombok?
We need to install Lombok in our system and integrate it with Eclipse IDE to use its annotations on the POJO class. The following steps of the Installation of Lombok are:
1. Get more details about Lombok from https://projectlombok.org/download
2. Click on that downloaded Lombok.jar file
3. If installed IDE paths are not showing automatically then you have to click on Specify a location to provide a path of IDEs

4. Click on the Install/Update button.
5. Go to Eclipse then click on About Eclipse IDE.

How to use the Lombok in the Spring Boot Application?
We need to create a Spring Boot Project and add the Lombok dependency on it. Here are the following steps for how to use Lombok in the Spring Boot Application. Let’s implement
1. Creating a Spring Boot Starter Project
2. Keep Eclipse IDE ready
3. Defining configurations
4. Creating a JPA Entity class
5. Create a JPA Repository
6. Create a Service
7. Inserting and Fetching Records
8. Run the Project and Check
1. Creating a Spring Boot Starter Project
We are creating a Spring Boot Project from the Spring Initializr web tool. You can create from your IDE which you are using.
Add the following dependencies:
• Spring Web
• Spring Data JPA
• Lombok
• H2 Database
Project Structure of How to Use Lombok in Spring Boot

Maven Dependency
Here is the complete pom.xml with Lombok dependency for this application.
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project
xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.6.3</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.poc</groupId>
<artifactId>Spring_Boot_Lombok_Proj</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>Spring_Boot_Lombok_Proj</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>11</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<excludes>
<exclude>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</exclude>
</excludes>
</configuration>
</plugin>
</plugins>
</build>
</project>
3. Defining configurations
We are defining the H2 database configuration in the application.properties file.
application.properties
spring.datasource.url=jdbc:h2:mem:test
#spring.datasource.driverClassName=org.h2.Driver
spring.datasource.username=sa
spring.datasource.password=
spring.jpa.show-sql=true
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.H2Dialect
spring.jpa.hibernate.ddl-auto= update
spring.h2.console.enabled=true
4. Creating a JPA Entity class
We are creating a JPA Entity class to create a table through Java code in the database. In this entity class, we will learn how to use Lombok to reduce boilerplate codes with its annotation.
Person.java
package com.poc.entity;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import lombok.Data;
@Entity
@Data
public class Person {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String fName;
private String lName;
}
→ @Getter and @Setter: Generates setter and getter methods.
→ @ToString: Generates toString method
→ @NoArgsConstructor and @AllArgsConstructor: Generates constructors that take no argument and one argument for every field.
→ @EqualsAndHashCode: Generates hashCode and equals implementations from the fields of your object.
→ @RequiredArgsConstructor: Generates one argument per final / non-null field.
→ @Data: A shortcut for @ToString, @EqualsAndHashCode, @Getter on all fields, and @Setter on all non-final fields and @RequiredArgsConstructor.
5. Creating a JPA Repository
We are creating a JPA Repository to communicate with the table and use the query method for persistence operations. This PersonRepository is extending the JpaRepository interface from Spring Data JPA.
PersonRepository.java
package com.poc.repo;
import org.springframework.data.jpa.repository.JpaRepository;
import com.poc.entity.Person;
public interface PersonRepository extends JpaRepository<Person, Long> {
}
6. Creating a Service
We are creating a service interface PersonService in which declaring two methods(save() and getPersonList()).
PersonService.java
package com.poc.service;
import java.util.List;
import com.poc.entity.Person;
public interface PersonService {
void save(Person person);
List<Person> getPersonList();
}
We are creating a class PersonServiceImpl that implements PersonService and this class is annotated with @Service annotation.
PersonServiceImpl.java
package com.poc.service;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.poc.entity.Person;
import com.poc.repo.PersonRepository;
@Service
public class PersonServiceImpl implements PersonService {
@Autowired
PersonRepository personRepo;
@Override
public void save(Person person) {
personRepo.save(person);
}
@Override
public List<Person> getPersonList() {
return personRepo.findAll();
}
}
7. Inserting and Fetching Records
We are inserting some records and fetching them from this class by using CommandLineRunner.
package com.poc;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.Bean;
import com.poc.entity.Person;
import com.poc.service.PersonService;
@SpringBootApplication
public class SpringBootJpaEntityPocApplication {
public static void main(String[] args) {
SpringApplication.run(SpringBootJpaEntityPocApplication.class, args);
}
@Bean
public CommandLineRunner demo(PersonService personService) {
return (args) -> {
// save few person
Person person1 = new Person();
person1.setfName("Peter");
person1.setlName("Parker");
Person person2 = new Person();
person2.setfName("Robert");
person2.setlName("Smith");
personService.save(person1);
personService.save(person2);
// fetch all person
System.out.println("-----List of Persons------");
for (Person person : personService.getPersonList()) {
System.out.println("Person Detail:" + person);
}
};
}
}
8. Run the Project and Check
→ Right-click on the java class(SpringBootLombokPocApplication.java) then click on Run As Java Application.
→ Type this URL http://localhost:8080/h2-console on the browser for the checking of the H2 database console.
To read more about using Lombok click here.
Conclusion
This topic is explained What is the Lombok? How to Add Lombok Dependency? How to use the Lombok in the Spring Boot Application? How to install the Lombok in our system?