Last updated on April 8th, 2024
We need to create a Spring Boot Application and add Spring Data JPA dependency on the pom.xml file on the application. Then create a JPA Entity class and create an interface that extends the JpaRepository interface. This JpaRepository interface is available in the Spring Data JPA library. In this topic, we will learn about What is JpaRepository, What is Spring Data JPA and how to use Jpa Repository in Spring Boot Application.

What is Spring Data JPA?
Spring Data JPA is a module of Spring Data that makes it easy for developers to implement JPA(Java Persistence API) repositories. It is very useful to store and retrieve data from the database through Java code without the need to write the query. JpaRepository is provided by the Spring Data JPA. That’s why we must add this dependency in the Spring Boot Application.
Maven Dependency of Spring Data JPA
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
What is JpaRepository?
A JpaRepository is an interface that extends the JPA Repository. It imports from the “org.springframework.data.jpa.repository” package. It is very useful for persistence operations. It provides us with query methods to perform persistence operations through Java code without writing manual queries.
Syntax
public interface JpaRepository<T, ID> extends PagingAndSortingRepository<T, ID>, QueryByExampleExecutor<T>
- T: It is a domain type that the repository manages (Entity class).
- ID: It is a type of the id of the entity class that the repository manages (Data type of Entity class’s @Id property).
Example
public interface EmployeeRepository extends JpaRepository<Employee, Long> {}
Methods
Some of the important methods available in the JpaRepository are commonly used. These are the following:
- save(): This method saves the given entity
Syntax
<S extends T> S save(S entity)
The parameter entity must be not null and return a saved entity.
- findById(): This method fetches an entity by id.
Syntax
Optional<T> findById(ID id)
The parameter id must be not null and returns an entity with the provided id.
- findAll(): This method fetches a list of entities.
Syntax
Iterable<T> findAll()
It returns all entities.
- delete(): This method deletes an entity.
Syntax
void delete(T entity)
Parameter an entity must not be null.
We will create a restful web service Spring Boot Application using Spring Web, Maven, Spring Data JPA, Lombok and H2 database step-by-step. Let’s implement
1. Creating a Spring Boot Starter Project
We are creating a Spring Boot Application from the web tool Spring Initializr or you can create it from the IDE(STS, VS Code etc.) you are using.
Add the following dependencies:
- Spring Web
- Spring Data JPA
- Lombok
- H2 Database
2. Keep the IDE ready
We are importing this created application into our Eclipse IDE or you can import it into another IDE you are using. You can refer to this article to create and set up the Spring Boot Project in Eclipse IDE.
Project Structure

3. Maven Dependency
Here is the complete pom.xml file for the Spring Boot Application.
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project
xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.6.3</version>
<relativePath />
<!-- lookup parent from repository -->
</parent>
<groupId>com.springjava</groupId>
<artifactId>demo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>demo</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
4. Defining the configuration
We are configuring the H2 database configuration in the application.properties file.
application.properties
# H2 Database Configuration
spring.datasource.url=jdbc:h2:mem:test
spring.datasource.username=sa
spring.datasource.password=
spring.jpa.show-sql=true
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.H2Dialect
spring.jpa.hibernate.ddl-auto=update
spring.h2.console.enabled=true
5. Creating a JPA Entity
We are creating a JPA entity class Employee with these properties(id, name, email, and mobileNo).
Employee.java
package com.springjava.entity;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import lombok.Data;
@Data
@Entity
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String email;
private String mobileNo;
}
- This @Data annotation is used for a constructor, setter method, getter method etc.
- This @Entity annotation is used to create a table through Java code in the database.
6. Creating a JPA Repository
We are a JPA Repository to interact with the JPA Entity class and use the query method for the persistence operation in the table employee.
EmployeeRepository.java
package com.springjava.repository;
import org.springframework.data.jpa.repository.JpaRepository;
import com.springjava.entity.Employee;
public interface EmployeeRepository extends JpaRepository < Employee, Long > {}
7. Creating a Service Interface
We are creating a Service interface with some method declaration(save(), findAll(), and findById()).
EmployeeService.java
package com.springjava.service;
import java.util.List;
import com.springjava.entity.Employee;
public interface EmployeeService {
void save(Employee employee);
List < Employee > findAll();
Employee findById(Long id);
void delete(Employee employee);
}
8. Creating a Service class
We are creating a Service class EmployeeServiceImpl and this class is implementing the EmployeeService interface. This class is annotated with @Service annotation to act service for the application.
EmployeeServiceImpl.java
package com.springjava.service;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.springjava.entity.Employee;
import com.springjava.repository.EmployeeRepository;
@Service
public class EmployeeServiceImpl implements EmployeeService {
@Autowired
EmployeeRepository empRepo;
@Override
public void save(Employee employee) {
empRepo.save(employee);
}
@Override
public List<Employee> findAll() {
return empRepo.findAll();
}
@Override
public Employee findById(Long id) {
return empRepo.findById(id).get();
}
@Override
public void delete(Employee employee) {
empRepo.delete(employee);
}
}
9. Creating a Rest Controller class
We are creating a RestController class EmployeeController in which all methods are created for API endpoints
EmployeeController.Java
package com.springjava.controller;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.DeleteMapping;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.PutMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import com.springjava.entity.Employee;
import com.springjava.service.EmployeeService;
@RestController
@RequestMapping("/api/employee")
public class EmployeeController {
@Autowired
EmployeeService empService;
@PostMapping("/save")
public ResponseEntity < ? > saveEmployee(@RequestBody Employee employee) {
Map < String, Object > respEmp = new LinkedHashMap < String, Object > ();
empService.save(employee);
respEmp.put("status", 1);
respEmp.put("message", "Record is Saved Successfully!");
return new ResponseEntity < > (respEmp, HttpStatus.CREATED);
}
@GetMapping("/list")
public ResponseEntity < ? > getEmployees() {
Map < String, Object > respEmp = new LinkedHashMap < String, Object > ();
List < Employee > empList = empService.findAll();
if (!empList.isEmpty()) {
respEmp.put("status", 1);
respEmp.put("data", empList);
return new ResponseEntity < > (respEmp, HttpStatus.OK);
} else {
respEmp.clear();
respEmp.put("status", 0);
respEmp.put("message", "Data is not found");
return new ResponseEntity < > (respEmp, HttpStatus.NOT_FOUND);
}
}
@GetMapping("/{id}")
public ResponseEntity < ? > getEmployeeById(@PathVariable Long id) {
Map < String, Object > respEmp = new LinkedHashMap < String, Object > ();
try {
Employee emp = empService.findById(id);
respEmp.put("status", 1);
respEmp.put("data", emp);
return new ResponseEntity < > (respEmp, HttpStatus.OK);
} catch (Exception ex) {
respEmp.clear();
respEmp.put("status", 0);
respEmp.put("message", "Data is not found");
return new ResponseEntity < > (respEmp, HttpStatus.NOT_FOUND);
}
}
@PutMapping("/update/{id}")
public ResponseEntity < ? > updateEmployeeById(@PathVariable Long id, @RequestBody Employee employee) {
Map < String, Object > respEmp = new LinkedHashMap < String, Object > ();
try {
Employee emp = empService.findById(id);
emp.setName(employee.getName());
emp.setEmail(employee.getEmail());
emp.setMobileNo(employee.getMobileNo());
empService.save(emp);
respEmp.put("status", 1);
respEmp.put("data", empService.findById(id));
return new ResponseEntity < > (respEmp, HttpStatus.OK);
} catch (Exception ex) {
respEmp.clear();
respEmp.put("status", 0);
respEmp.put("message", "Data is not found");
return new ResponseEntity < > (respEmp, HttpStatus.NOT_FOUND);
}
}
@DeleteMapping("/delete/{id}")
public ResponseEntity < ? > deleteEmployee(@PathVariable Long id) {
Map < String, Object > respEmp = new LinkedHashMap < String, Object > ();
try {
Employee emp = empService.findById(id);
empService.delete(emp);
respEmp.put("status", 1);
respEmp.put("message", "Record is deleted successfully!");
return new ResponseEntity < > (respEmp, HttpStatus.OK);
} catch (Exception ex) {
respEmp.clear();
respEmp.put("status", 0);
respEmp.put("message", "Data is not found");
return new ResponseEntity < > (respEmp, HttpStatus.NOT_FOUND);
}
}
}
10. Run the Spring Boot Application and Check
Right Click on the DemoApplication.java then click on Run As, and select Java Application.
Check H2 Database
Check the H2 database console and browse this URL “http://localhost:8080/h2-console”.
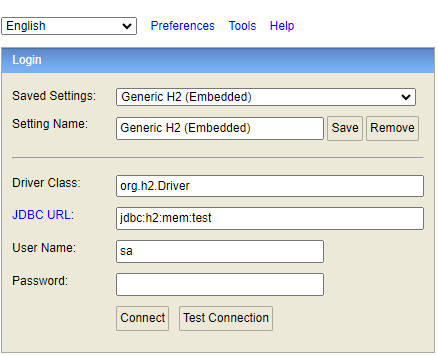
See the below table here:
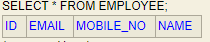
Testing API on the Postman
Saving the employee data
POST: http://localhost:8080/api/employee/save
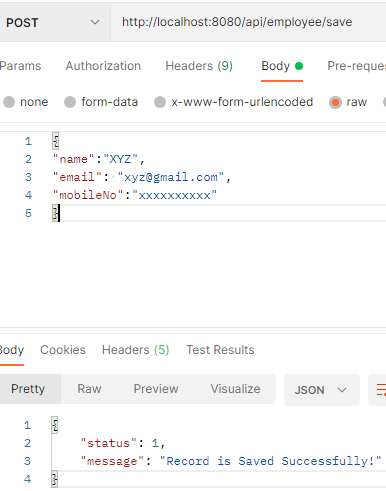
Check the table:

Retrieving the employee data
GET: http://localhost:8080/api/employee/list
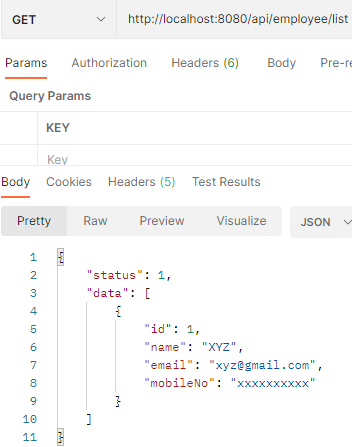
Retrieving the employee data by Id
GET: http://localhost:8080/api/employee/1
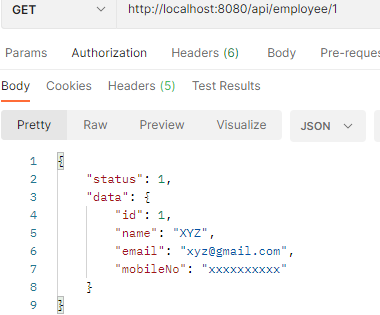
Updating the employee by Id
PUT: http://localhost:8080/api/employee/update/1

Deleting the employee data by Id
DELETE: http://localhost:8080/api/employee/delete/1

Check the table:

Conclusion
In this topic, we learnt about what is Spring Data JPA, What is JpaRepository and how to use JpaRepository in Spring Boot Rest Application.